The high-level steps to initiate a Process from a Project in Visual Studio are:
(1) Add a Web Reference to the Initiator.ashx file, based upon the URL
(2) Create a reference to the Service
a. Instantiate the Solution Data Items
b. Instantiate the Process Data Items
(3) Generate or Assign Tracking Identifiers as appropriate
a. Process Instance ID
b. Runspace ID
(4) Populate Data Items
a. Solution Data Items, a.k.a. StartData.DataItems
b. Process Data Items, a.k.a. StartData.DataItems1
(5) Set Credentials as appropriate
(6) Initiate the Process
Let’s look at an example process with the following components:
· A Data Model with two elements named: Employee
o Text Element: Email
o Text Element: Name
· Two Solution Data Items
o Simple Data, DateTime: LeadSubmittedDateTime
o Modeled Data (not a Collection) using Employee data model: Manager
· Two Process Data Items
o Simple Data, Text: LeadNumber
o Collection of Modeled Data using Employee data model: SalesPeople
Create a new web reference named SalesProcess. Set the web reference to the Initiator.ashx file based on the URL as described previously. You could then setup the following method to launch a process:
public void InitiateProcess()
{
using (SalesProcess.Start service = new SalesProcess.Start())
{
// Generate solution and process tracking GUIDs
string runspaceID = Guid.NewGuid().ToString();
string processInstanceID = Guid.NewGuid().ToString();
// Initialize startData (data items) object
SalesProcess.StartData startData = new SalesProcess.StartData();
// Instantiate Solution Data Items
startData.DataItems = new SalesProcess.SolutionDataItems();
// Populate a simple Solution Data Item named LeadSubmittedDateTime
startData.DataItems.LeadSubmittedDateTime = new DateTime(2012, 7, 17, 11, 56, 45, 128);
// Populate a Data Model Solution Data Item named Manager
// The modeled solution data item is based on the data model named Employee
startData.DataItems.Manager = new SalesProcess.Employee();
startData.DataItems.Manager.Name = "Jane Herin";
startData.DataItems.Manager.Email = "jane.herin@bluespringsoftware.com";
// Instantiate Process Data Items
startData.DataItems1 = new SalesProcess.ProcessDataItems();
// Populate a simple Process Data Item named LeadNumber
startData.DataItems1.LeadNumber = "101583";
// Populate a Collection Process Data Item named SalesPeople
// The collection data item is based on the data model named Employee
startData.DataItems1.SalesPeople = new SalesProcess.Employee[] {
new SalesProcess.Employee() {Email="alan.summers@bluespringsoftware.com", Name="Alan Summers"},
new SalesProcess.Employee() {Email="joe.newbody@bluespringsoftware.com", Name="Joe Newbody"}
};
// Set credentials for the service
service.UseDefaultCredentials = true;
// Start the process
service.CallStart(runspaceID, processInstanceID, startData);
}
}
When the above InitiateProcess() method is called, we can look in BPM Admin and see that a process has been launched with the Data Items populated as expected:
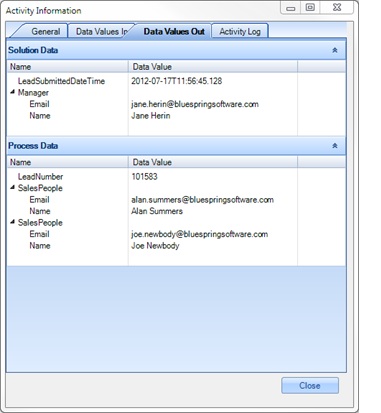